Tricking Mapbox with synthetic events
Creating and dispatching custom DOM events
The Problem
If you overlay HTML on top of a
Potential Solutions
1. Map forwards gestures to overlayed
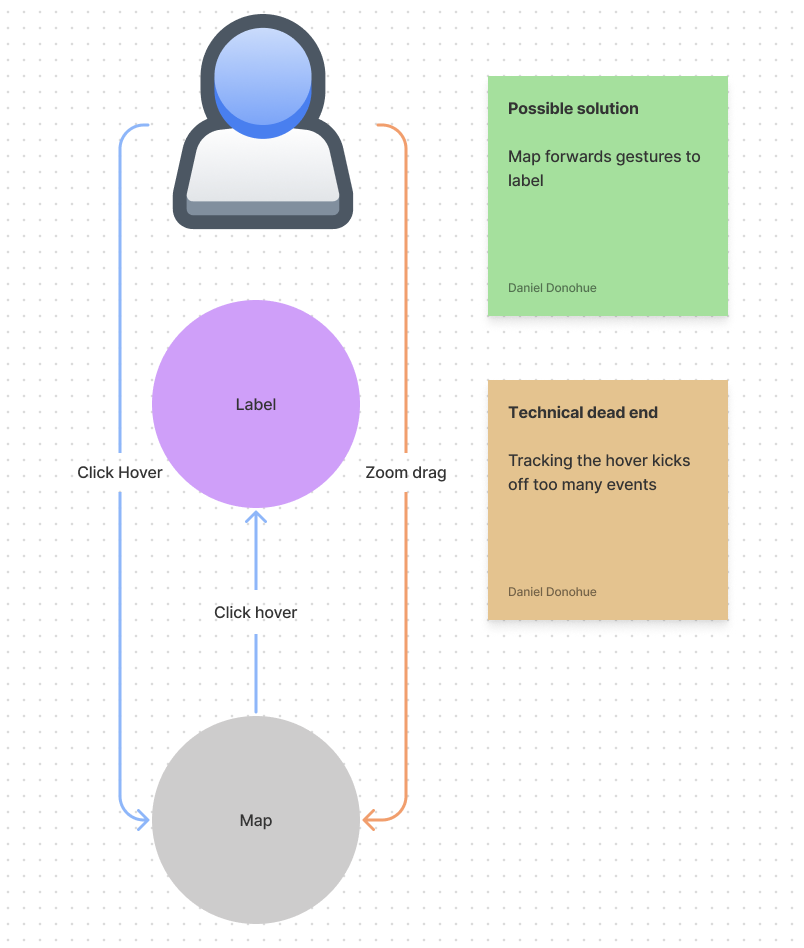
We would remove all events from the
The con of this solution were the expensive event listeners. Since we have to constantly track the position of the mouse and check if it was on our
2. The label captures hovers and clicks, while the map handles pan and zooms
It would be nice if we could have found a way to turn off some events, rather than all like with the pointer-events: none. However, from what we saw this was not possible.
Our Solution
The label forwards events to the map.
We create events within the label using the Event Constructor then dispatch them ON the
const canvas = document.querySelector('#map'); const label = document.querySelector('#label'); label.addEventListener('mousedown', e => { let options = { pageX: e.pageX, pageY: e.pageY, clientX: e.clientX, clientY: e.clientY, }; canvas.dispatchEvent(new MouseEvent('mousedown', options)); });
Find both the map and label from the DOM, add new event listener for mouse down to the label, dispatch a mouse event with the relevant fields to the canvas and 💥, you have drag panning on the map while mousedowned on a